Macos Create Terminal App Tutorial
First, we create the user 'container' for the account: sudo dscl.create /Users/sally Next, we set the user's shell. We use bash in this example. The macOS default is /bin/tcsh and the most recommended shell is /bin/zsh.create /Users/sally UserShell /bin/bash Next, we set the actual name of. First, a macOS running on a virtual machine or an actual Mac and second a reliable internet connection for downloading macOS High Sierra from the App Store which will be then converted to ISO. If you’re one of those users who have these services in hand, we could likely show how to Create macOS.
In this tutorial, you'll build a Flask web app that uses Azure Cognitive Services to translate text, analyze sentiment, and synthesize translated text into speech. Our focus is on the Python code and Flask routes that enable our application, however, we will help you out with the HTML and Javascript that pulls the app together. If you run into any issues let us know using the feedback button below.
Here's what this tutorial covers:
- Get Azure subscription keys
- Set up your development environment and install dependencies
- Create a Flask app
- Use the Translator to translate text
- Use Text Analytics to analyze positive/negative sentiment of input text and translations
- Use Speech Services to convert translated text into synthesized speech
- Run your Flask app locally
Tip
If you'd like to skip ahead and see all the code at once, the entire sample, along with build instructions are available on GitHub.
What is Flask?
Flask is a microframework for creating web applications. This means Flask provides you with tools, libraries, and technologies that allow you to build a web application. This web application can be some web pages, a blog, a wiki or go as substantive as a web-based calendar application or a commercial website.
For those of you who want to deep dive after this tutorial here are a few helpful links:
Prerequisites
Let's review the software and subscription keys that you'll need for this tutorial.
- An IDE or text editor, such as Visual Studio Code or Atom
- Chrome or Firefox
- A Translator subscription key (Note that you aren't required to select a region.)
- A Text Analytics subscription key in the West US region.
- A Speech Services subscription key in the West US region.
Create an account and subscribe to resources
As previously mentioned, you're going to need three subscription keys for this tutorial. This means that you need to create a resource within your Azure account for:
- Translator
- Text Analytics
- Speech Services
Use Create a Cognitive Services Account in the Azure portal for step-by-step instructions to create resources.
Important
For this tutorial, please create your resources in the West US region. If using a different region, you'll need to adjust the base URL in each of your Python files.
Set up your dev environment
Before you build your Flask web app, you'll need to create a working directory for your project and install a few Python packages.
Create a working directory
Open command line (Windows) or terminal (macOS/Linux). Then, create a working directory and sub directories for your project:
Change to your project's working directory:
Create and activate your virtual environment with virtualenv
Let's create a virtual environment for our Flask app using virtualenv
. Using a virtual environment ensures that you have a clean environment to work from.
In your working directory, run this command to create a virtual environment:macOS/Linux:
We've explicitly declared that the virtual environment should use Python 3. This ensures that users with multiple Python installations are using the correct version.
Windows CMD / Windows Bash:
To keep things simple, we're naming your virtual environment venv.
The commands to activate your virtual environment will vary depending on your platform/shell:
Platform Shell Command macOS/Linux bash/zsh source venv/bin/activate
Windows bash source venv/Scripts/activate
Command Line venvScriptsactivate.bat
PowerShell venvScriptsActivate.ps1
After running this command, your command line or terminal session should be prefaced with
venv
.You can deactivate the session at any time by typing this into the command line or terminal:
deactivate
.
Note
Python has extensive documentation for creating and managing virtual environments, see virtualenv.
Macos App Develop
Install requests
Requests is a popular module that is used to send HTTP 1.1 requests. There's no need to manually add query strings to your URLs, or to form-encode your POST data.
To install requests, run:
Note
If you'd like to learn more about requests, see Requests: HTTP for Humans.
Install and configure Flask
Next we need to install Flask. Flask handles the routing for our web app, and allows us to make server-to-server calls that hide our subscription keys from the end user.
To install Flask, run:
Let's make sure Flask was installed. Run:
The version should be printed to terminal. Anything else means something went wrong.
To run the Flask app, you can either use the flask command or Python's -m switch with Flask. Before you can do that you need to tell your terminal which app to work with by exporting the
FLASK_APP
environment variable:macOS/Linux:
Windows:
Create your Flask app
In this section, you're going to create a barebones Flask app that returns an HTML file when users hit the root of your app. Don't spend too much time trying to pick apart the code, we'll come back to update this file later.
What is a Flask route?
Let's take a minute to talk about 'routes'. Routing is used to bind a URL to a specific function. Flask uses route decorators to register functions to specific URLs. For example, when a user navigates to the root (/
) of our web app, index.html
is rendered.
Let's take a look at one more example to hammer this home.
This code ensures that when a user navigates to http://your-web-app.com/about
that the about.html
file is rendered.
While these samples illustrate how to render html pages for a user, routes can also be used to call APIs when a button is pressed, or take any number of actions without having to navigate away from the homepage. You'll see this in action when you create routes for translation, sentiment, and speech synthesis.
Get started
Open the project in your IDE, then create a file named
app.py
in the root of your working directory. Next, copy this code intoapp.py
and save:This code block tells the app to display
index.html
whenever a user navigates to the root of your web app (/
).Next, let's create the front-end for our web app. Create a file named
index.html
in thetemplates
directory. Then copy this code intotemplates/index.html
.Let's test the Flask app. From the terminal, run:
Open a browser and navigate to the URL provided. You should see your single page app. Press Ctrl + C to kill the app.
Translate text
Now that you have an idea of how a simple Flask app works, let's:

- Write some Python to call the Translator and return a response
- Create a Flask route to call your Python code
- Update the HTML with an area for text input and translation, a language selector, and translate button
- Write Javascript that allows users to interact with your Flask app from the HTML
Call the Translator
The first thing you need to do is write a function to call the Translator. This function will take two arguments: text_input
and language_output
. This function is called whenever a user presses the translate button in your app. The text area in the HTML is sent as the text_input
, and the language selection value in the HTML is sent as language_output
.
- Let's start by creating a file called
translate.py
in the root of your working directory. - Next, add this code to
translate.py
. This function takes two arguments:text_input
andlanguage_output
. - Add your Translator subscription key and save.
Add a route to app.py
Next, you'll need to create a route in your Flask app that calls translate.py
. This route will be called each time a user presses the translate button in your app.
For this app, your route is going to accept POST
requests. This is because the function expects the text to translate and an output language for the translation.
Flask provides helper functions to help you parse and manage each request. In the code provided, get_json()
returns the data from the POST
request as JSON. Then using data['text']
and data['to']
, the text and output language values are passed to get_translation()
function available from translate.py
. The last step is to return the response as JSON, since you'll need to display this data in your web app.
In the following sections, you'll repeat this process as you create routes for sentiment analysis and speech synthesis.
Macos Create Terminal App Tutorial Download
Open
app.py
and locate the import statement at the top ofapp.py
and add the following line:Now our Flask app can use the method available via
translate.py
.Copy this code to the end of
app.py
and save:
Update index.html
Now that you have a function to translate text, and a route in your Flask app to call it, the next step is to start building the HTML for your app. The HTML below does a few things:
- Provides a text area where users can input text to translate.
- Includes a language selector.
- Includes HTML elements to render the detected language and confidence scores returned during translation.
- Provides a read-only text area where the translation output is displayed.
- Includes placeholders for sentiment analysis and speech synthesis code that you'll add to this file later in the tutorial.
Let's update index.html
.
Open
index.html
and locate these code comments:Replace the code comments with this HTML block:
The next step is to write some Javascript. This is the bridge between your HTML and Flask route.
Create main.js
The main.js
file is the bridge between your HTML and Flask route. Your app will use a combination of jQuery, Ajax, and XMLHttpRequest to render content, and make POST
requests to your Flask routes.
In the code below, content from the HTML is used to construct a request to your Flask route. Specifically, the contents of the text area and the language selector are assigned to variables, and then passed along in the request to translate-text
.
The code then iterates through the response, and updates the HTML with the translation, detected language, and confidence score.
- From your IDE, create a file named
main.js
in thestatic/scripts
directory. - Copy this code into
static/scripts/main.js
:
Test translation
Let's test translation in the app.
Navigate to the provided server address. Type text into the input area, select a language, and press translate. You should get a translation. If it doesn't work, make sure that you've added your subscription key.
Tip
If the changes you've made aren't showing up, or the app doesn't work the way you expect it to, try clearing your cache or opening a private/incognito window.
Press CTRL + c to kill the app, then head to the next section.
Analyze sentiment
The Text Analytics API can be used to perform sentiment analysis, extract key phrases from text, or detect the source language. In this app, we're going to use sentiment analysis to determine if the provided text is positive, neutral, or negative. The API returns a numeric score between 0 and 1. Scores close to 1 indicate positive sentiment, and scores close to 0 indicate negative sentiment.
In this section, you're going to do a few things:
- Write some Python to call the Text Analytics API to perform sentiment analysis and return a response
- Create a Flask route to call your Python code
- Update the HTML with an area for sentiment scores, and a button to perform analysis
- Write Javascript that allows users to interact with your Flask app from the HTML
Call the Text Analytics API
Let's write a function to call the Text Analytics API. This function will take four arguments: input_text
, input_language
, output_text
, and output_language
. This function is called whenever a user presses the run sentiment analysis button in your app. Data provided by the user from the text area and language selector, as well as the detected language and translation output are provided with each request. The response object includes sentiment scores for the source and translation. In the following sections, you're going to write some Javascript to parse the response and use it in your app. For now, let's focus on call the Text Analytics API.
- Let's create a file called
sentiment.py
in the root of your working directory. - Next, add this code to
sentiment.py
. - Add your Text Analytics subscription key and save.
Add a route to app.py
Let's create a route in your Flask app that calls sentiment.py
. This route will be called each time a user presses the run sentiment analysis button in your app. Like the route for translation, this route is going to accept POST
requests since the function expects arguments.
Open
app.py
and locate the import statement at the top ofapp.py
and update it:Now our Flask app can use the method available via
sentiment.py
.Copy this code to the end of
app.py
and save:
Update index.html
Now that you have a function to run sentiment analysis, and a route in your Flask app to call it, the next step is to start writing the HTML for your app. The HTML below does a few things:
- Adds a button to your app to run sentiment analysis
- Adds an element that explains sentiment scoring
- Adds an element to display the sentiment scores
Open
index.html
and locate these code comments:Replace the code comments with this HTML block:
Update main.js
In the code below, content from the HTML is used to construct a request to your Flask route. Specifically, the contents of the text area and the language selector are assigned to variables, and then passed along in the request to the sentiment-analysis
route.
The code then iterates through the response, and updates the HTML with the sentiment scores.
From your IDE, create a file named
main.js
in thestatic
directory.Copy this code into
static/scripts/main.js
:
Test sentiment analysis
Let's test sentiment analysis in the app.
Navigate to the provided server address. Type text into the input area, select a language, and press translate. You should get a translation. Next, press the run sentiment analysis button. You should see two scores. If it doesn't work, make sure that you've added your subscription key.
Tip
If the changes you've made aren't showing up, or the app doesn't work the way you expect it to, try clearing your cache or opening a private/incognito window.
Press CTRL + c to kill the app, then head to the next section.
Convert text-to-speech
The Text-to-speech API enables your app to convert text into natural human-like synthesized speech. The service supports standard, neural, and custom voices. Our sample app uses a handful of the available voices, for a full list, see supported languages.
In this section, you're going to do a few things:
- Write some Python to convert text-to-speech with the Text-to-speech API
- Create a Flask route to call your Python code
- Update the HTML with a button to convert text-to-speech, and an element for audio playback
- Write Javascript that allows users to interact with your Flask app
Call the Text-to-Speech API
Let's write a function to convert text-to-speech. This function will take two arguments: input_text
and voice_font
. This function is called whenever a user presses the convert text-to-speech button in your app. input_text
is the translation output returned by the call to translate text, voice_font
is the value from the voice font selector in the HTML.
Let's create a file called
synthesize.py
in the root of your working directory.Next, add this code to
synthesize.py
.Add your Speech Services subscription key and save.
Add a route to app.py
Let's create a route in your Flask app that calls synthesize.py
. This route will be called each time a user presses the convert text-to-speech button in your app. Like the routes for translation and sentiment analysis, this route is going to accept POST
requests since the function expects two arguments: the text to synthesize, and the voice font for playback.
Open
app.py
and locate the import statement at the top ofapp.py
and update it:Now our Flask app can use the method available via
synthesize.py
.Copy this code to the end of
app.py
and save:
Update index.html
Now that you have a function to convert text-to-speech, and a route in your Flask app to call it, the next step is to start writing the HTML for your app. The HTML below does a few things: Best photo editing software for mac reviews.
- Provides a voice selection drop-down
- Adds a button to convert text-to-speech
- Adds an audio element, which is used to play back the synthesized speech
Open
index.html
and locate these code comments:Replace the code comments with this HTML block:
Next, locate these code comments:
Replace the code comments with this HTML block:
- Make sure to save your work.
Update main.js
In the code below, content from the HTML is used to construct a request to your Flask route. Specifically, the translation and the voice font are assigned to variables, and then passed along in the request to the text-to-speech
route.
The code then iterates through the response, and updates the HTML with the sentiment scores.
- From your IDE, create a file named
main.js
in thestatic
directory. - Copy this code into
static/scripts/main.js
: - You're almost done. The last thing you're going to do is add some code to
main.js
to automatically select a voice font based on the language selected for translation. Add this code block tomain.js
:
Test your app
Let's test speech synthesis in the app.
Navigate to the provided server address. Type text into the input area, select a language, and press translate. You should get a translation. Next, select a voice, then press the convert text-to-speech button. the translation should be played back as synthesized speech. If it doesn't work, make sure that you've added your subscription key.
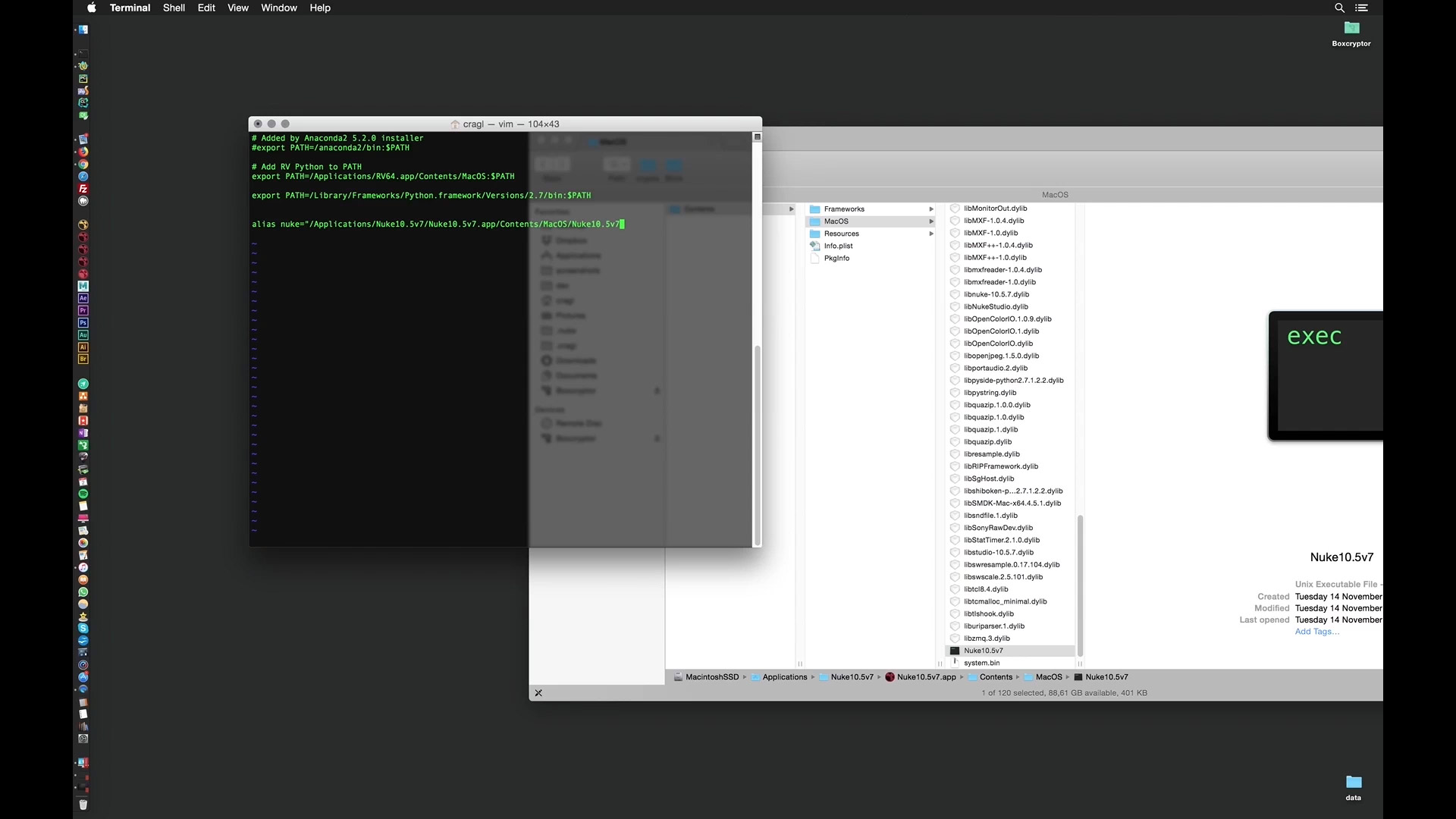
Tip
If the changes you've made aren't showing up, or the app doesn't work the way you expect it to, try clearing your cache or opening a private/incognito window.
That's it, you have a working app that performs translations, analyzes sentiment, and synthesized speech. Press CTRL + c to kill the app. Be sure to check out the other Azure Cognitive Services.
Get the source code
The source code for this project is available on GitHub.